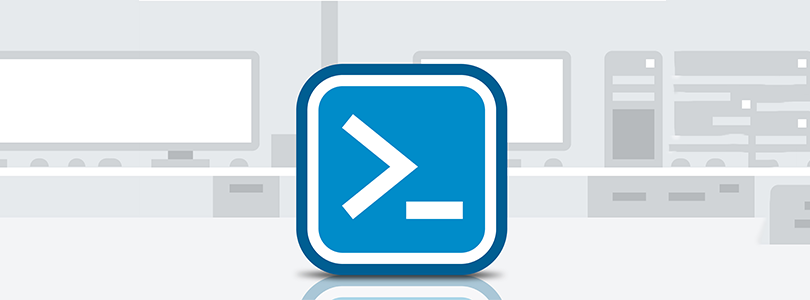
Quick post on how to connect to Microsoft Graph API with
PowerShell. Microsoft
Graph API is different from Azure
Active Directory Graph API. The fastest way to tell them apart is by
looking at the URL
Microsoft Graph API
- https://graph.microsoft.com
Azure Active
Directory Graph API - https://graph.windows.net
You will need Azure Resource Manager Module. More information on how to install AzureRM:
AzureRM 4.4.0
Install and configure Azure PowerShell
AzureRM 4.4.0
Install and configure Azure PowerShell
To connect to Microsoft Graph API you need to register an
App in AzureAD
· Login
to https://portal.azure.com and navigate to Azure Active Directory > App
Registrations and click New
Application Registration
· Input
a Name, select application type as Native,
and enter a redirect URL in the format urn:ReportingURN,
click create. Note: For this script we do not have a specific requirement for
the Redirect URI, the exact values doesn't matter as long as it's in the format
URN:<Value>
· Click
on the App and choose Settings, navigate to Required Permissions, click Add and select the Microsoft Graph API
· Choose Select Permissions and grant the App the necessary permissions. For the example below you’ll need Read all usage reports
· Copy the Application ID and the URN
After that use the following PowerShell Function to get a
Token. Supply a tenant name (domain.com) and change the $clientID and $redirectURI
to the ones you got when registering the App
Function
GetAuthToken($tenantName){
Import-Module Azure
$clientId =
"a11118ab-7777-9999-1111-2222b2b3333c"
$redirectUri = "urn:appURN"
$resourceAppIdURI = "https://graph.microsoft.com"
$authority =
"https://login.microsoftonline.com/$tenantName"
$authContext = New-Object "Microsoft.IdentityModel.Clients.ActiveDirectory.AuthenticationContext"
-ArgumentList $authority
$Credential = Get-Credential
$AADCredential = New-Object "Microsoft.IdentityModel.Clients.ActiveDirectory.UserCredential"
-ArgumentList $credential.UserName,$credential.Password
$authResult = $authContext.AcquireToken($resourceAppIdURI, $clientId,$AADCredential)
return $authResult
}
Now you can access Microsoft Graph API. Here’s an example of
a function obtaining Exchange Usage Report:
Function
GetExchangeReport($tenant)
{
$token =
GetAuthToken -TenantName
$tenant
$authHeader = @{
'Content-Type'='application\json'
'Authorization'=$token.CreateAuthorizationHeader()
}
$uri =
"https://graph.microsoft.com/v1.0/reports/getEmailActivityCounts(period='D7')"
$report =
Invoke-RestMethod -Uri
$uri –Headers
$authHeader –Method
Get
return $report
}
Comments
Post a Comment